Coding in Java private int get
Coding in Java
- private int getLastDayOfMonth(int m, int y)– return the lastday of the month and year entered as input parameter. Remember toinvoke the isLeapYear method when appropriate. If the month enteredas input parameter is 2 (February) and the year passed as inputparameter is a leap year this method should return 29.
- private boolean isDateValid (int m, int d, int y) – return trueif day, month, and year entered as input parameters form a validdate, return false otherwise. To be able to write the logic forthis method you need to know the number of days for each month ofthe year, whether a year is a leap year (leap years have 29 daysfor the month of February, not leap years have 28 days for themonth of February). Remember to invoke the isLeapYear andgetLastDayOfMonth methods when appropriate. Valid months arebetween 1 and 12 (inclusive) and consider a positive number as avalid year.
Examples:
- Invalid dates:
- 2/29/2019 – 2019 is not a leap year, the number of days inFebruary is 28
- 13/17/2019 – 13 is not a valid month
- 11/31/2019 – the month of November only has 30 days
- 6/23/-2019 – the year cannot be negative
- Valid dates:
- 2/29/2020 – 2020 is a leap year, February has 29 days
- 11/30/2019 – nothing wrong, November has 30 days
- 6/22/1992 – nothing wrong here.
Answer:
I have implemented these method under a single classTest , so in order to use them(for getting output)i have made them static and used them under ourmain method.
Since there is no information about input, I have asked userinput to enter day, month ,year separately and then perform thetasks.(Please see output screenshots to get clarity).
Please go through the code, test run it for various inputs, Openfor suggestions, Thanks!
import java.util.Scanner; //to prompt for user input
public class Test {
public static void main(String[] args) { Scanner sc = newScanner(System.in);// to prompt user input System.out.print(“Enter month:”); int month = sc.nextInt();// storesmonth System.out.print(“Enter day:”); int day = sc.nextInt();// storesday System.out.print(“Enteryear:”); int year = sc.nextInt();// storesyear /* * method call isDateValid(m,d,y) tocheck if the date entered is valid or not */ if (isDateValid(month, day, year)){ System.out.println(month + “/” + day + “/” + year + ” ValidDate”);// prints if the date is valid } else { System.out.println(month + “/” + day + “/” + year + ” InvalidDate”);// prints if the date is invalid } sc.close(); } /*month, day, year are passed as argument to belowfunction to test whether it forms a valid date or not*/ private static boolean isDateValid(int month, int day,int year) { boolean isDateValid =false;//boolean to validate the date int lastDayOfMonth =getLastDayOfMonth(month, year);/*stores the last day of themonth(passed as arg)*/ /*checks if month, day, year arepositive values*/ if (month > 0 && day> 0 && year > 0) { /*if monthentered is invalid getLastDayOfMonth returns 0(look for methodimplementation)*/ if(lastDayOfMonth == 0) { /*returns false here since isValidDate wasinitialized as false*/ return isDateValid; } /*if its a validmonth then check for entered day as valid or not should be between1 and lastDayOfMonth*/ if (day >= 1&& day <= lastDayOfMonth) { isDateValid = true; return isDateValid;//returns true here sincedate is found valid } } return isDateValid;//returns falsehere for negative values of either of day, month, year } /*returns the last day of month and year passed asargument*/ private static int getLastDayOfMonth(int month, intyear) { /*if month is valid i.e between 1and 12*/ if ((month >= 1 && month<= 12)) { /*For monthsJanuary,March,May,July,August,October,December last day is31st*/ if (month == 1|| month == 3 || month == 5 || month == 7 || month == 8 || month ==10 || month == 12) { return 31; } /*For monthsSeptember,April,June,November last day is 30th*/ else if (month== 9 || month == 4 || month == 6 || month == 11) { return 30; } /*For monthFebruary we need to check if its a leap year or not*/ else { /*checks for year being a leap year or not andreturn 29 or 28 accordingly*/ if (isLeapYear(year)) { return 29; } return 28; } } /*returns 0 for invalid monthentered*/ return 0; } /*returns true if its a leap year and false ifnot*/ private static boolean isLeapYear(int year) { /*a leap year should be either bedivisible by 400 or divisible by 4 and not divisible by 100*/ if (((year % 4 == 0) &&(year % 100 != 0)) || (year % 400 == 0)) { returntrue;//returns true for a leap year } return false;//returns false if notleap year }
}
screenshot of the code:
output when you run above code(multiple runs):
to format your code Ctrl+a then Ctrl+Shift+f(for eclipseIDE)
"Our Prices Start at $11.99. As Our First Client, Use Coupon Code GET15 to claim 15% Discount This Month!!"
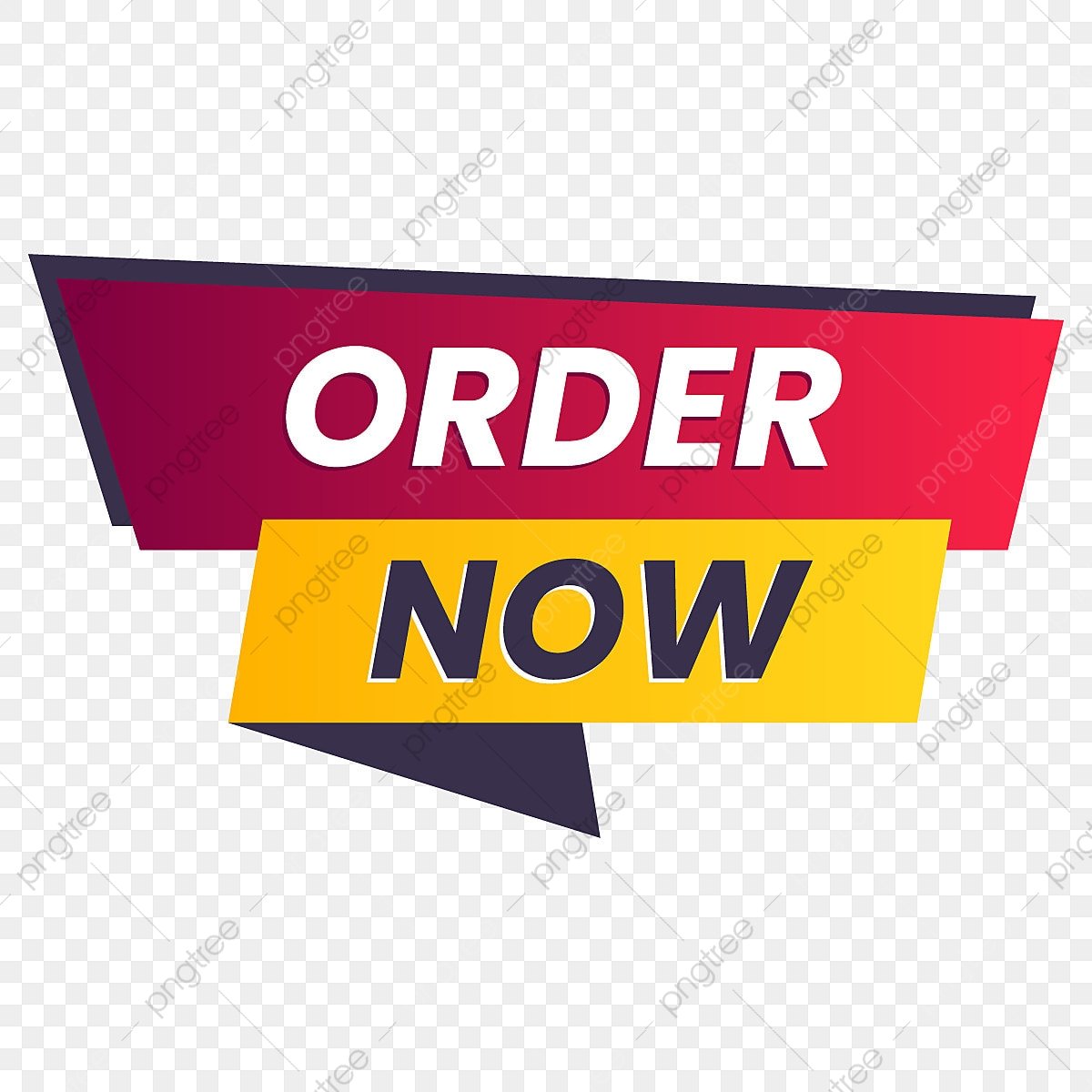