Using c++ Design a system to k
Using c++ Design a system to keep track of employee data. Thesystem should keep track of an employee’s name, ID number andhourly pay rate in a class calledEmployee.
You may also store any additional data you may need, (hint: youneed something extra). This data is stored in a file (userselectable) with the id number, hourly pay rate, and the employee’sfull name (example):
17 5.25 Daniel Katz18 6.75 John F. Jones
Start your main method by asking the user the name ofthe file to open.
Additionally we would like to be able to print payrollinformation from data in a different file. The data is theemployee’s id number and a number of hours that they worked(example):
17 4018 2018 20
As you see we can have the same number listed twice in whichcase that person should be paid the sum of the numbers (John Jonesdid 40 hours work, but it’s listed as 20+20).
You should start by reading in the first file and storing thedata as objects in a linked list. You will need tocreate the linked list class and the Employee data class.
You may choose to use the Linked List class we created, or youmay opt to create your own doubly linked list class. Do not use STLlinked class.The Linked list could be templated or not, it’s up toyou, however templating it would allow it to be used for otherprojects, so it might be a good idea.
Once you have read in the information from the first file, readin the second file. Ultimately we would like to print payrollinformation based on the hourly wage from the first file multipliedby the number of times an employee worked in the second file. Howyou do this is entirely up to you.
The output must be in sorted (descending; so the person who getspaid most prints first) order in the form of:
*********Payroll Information********Daniel Katz, $210John F. Jones, $270*********End payroll**************
The basic flow of the program is asfollows:
- Ask the user for the name of a file to open.
- Read in the employee information and to the list.
- Ask the user for the name of a file to open.
- Read in the payroll information
- Output the Payroll Information
Answer:
#include<iostream>#include<cstdlib>#include<fstream>#include<cstring>using namespace std;class Employee{string name[500];int id[500];float payrate[500];float payroll[500];int count;public:Employee(){count=0;}void readData(ifstream &f1,ifstream &f2){string str;while(getline(f1,str)){int i=1;string s;s=str[i-1];while(str[i]!=' '){s=s+str[i];i++;}id[count]=atoi(s.c_str());i=i+2;string s1;s1=str[i-1];while(str[i]!=' '){s1=s1+str[i];i++;}payrate[count]=atof(s1.c_str());i=i+2;name[count]=str[i-1];while(str[i]!='\0'){name[count]=name[count]+str[i];i++;}//char x;payroll[count]=0;count++;}int tid,temp;while(!f2.eof()){f2>>tid>>temp;for(int i=0;i<count;i++){if(tid==id[i]){payroll[i]=payroll[i]+temp*payrate[i];}}}}void printInfo(){cout<<endl;for(int i=0;i<count;i++){cout<<name[i]<<","<<payroll[i]<<endl;}}};int main(){Employee obj;char fname[100];cout<<"Enter file name:";ifstream f1;cin>>fname;f1.open(fname);if(!f1){cout<<"File opening error";return 0;}cout<<"Enter file name:";ifstream f2;cin>>fname;f2.open(fname);if(!f2){cout<<"File opening error";return 0;}obj.readData(f1,f2);obj.printInfo();return 0;}
"Our Prices Start at $11.99. As Our First Client, Use Coupon Code GET15 to claim 15% Discount This Month!!"
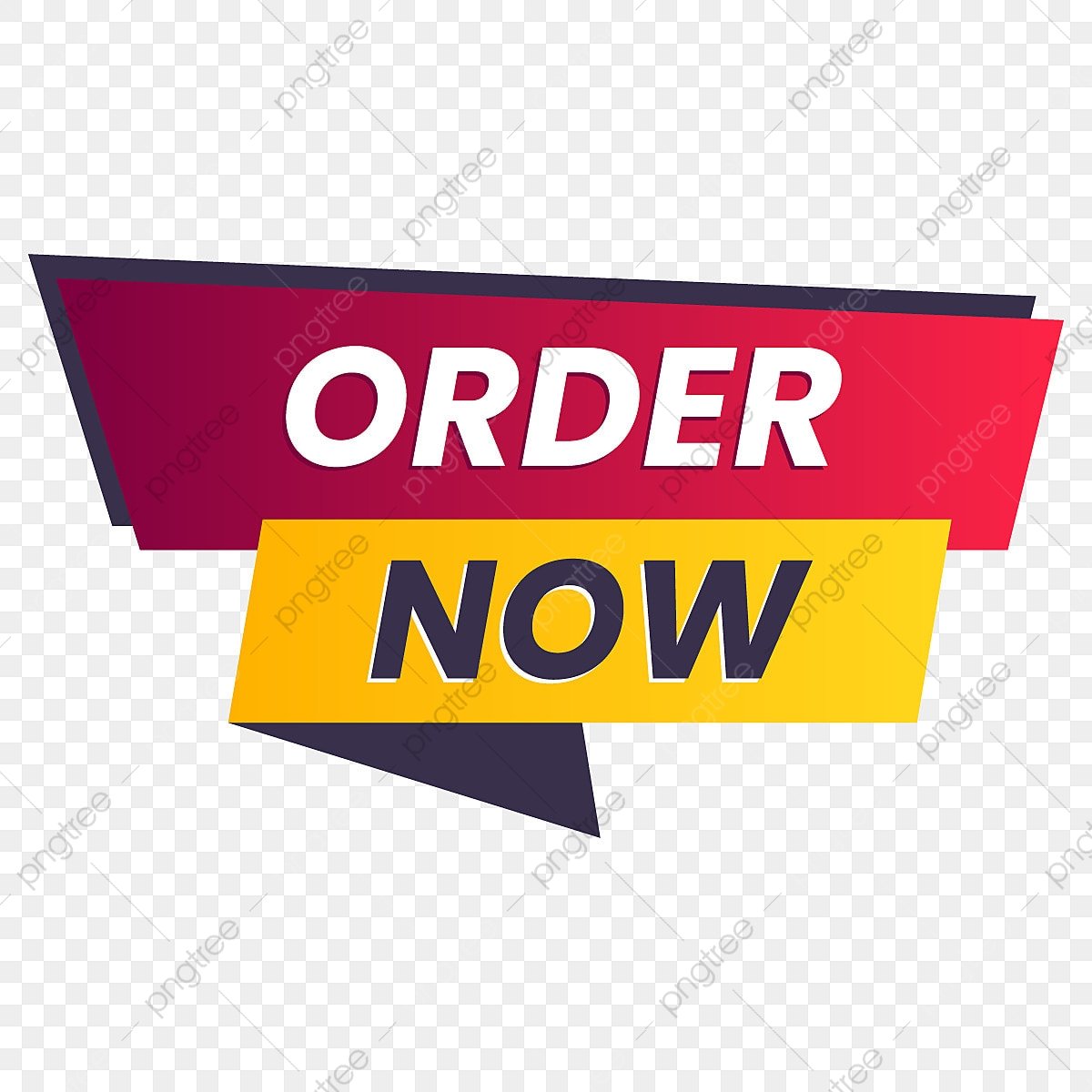